class mrpt::opengl::CPointCloud
Overview
A cloud of points, all with the same color or each depending on its value along a particular coordinate axis.
This class is just an OpenGL representation of a point cloud. For operating with maps of points, see mrpt::maps::CPointsMap and derived classes.
To load from a points-map, CPointCloud::loadFromPointsMap().
This class uses smart optimizations while rendering to efficiently draw clouds of millions of points, using octrees.
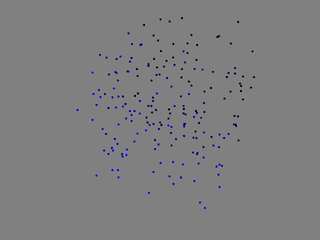
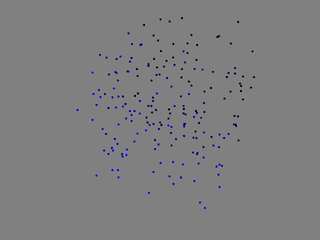
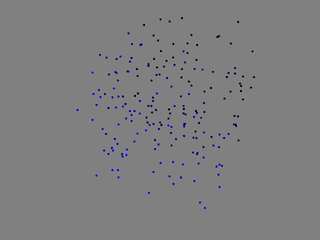
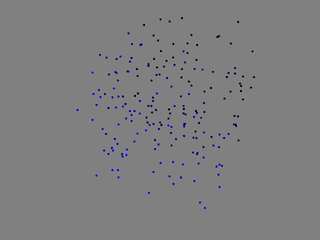
See also:
opengl::CPlanarLaserScan, opengl::Scene, opengl::CPointCloudColoured, mrpt::maps::CPointsMap
#include <mrpt/opengl/CPointCloud.h> class CPointCloud: public mrpt::opengl::CRenderizableShaderPoints, public mrpt::opengl::COctreePointRenderer, public mrpt::opengl::PLY_Importer, public mrpt::opengl::PLY_Exporter { public: // construction CPointCloud(); // methods size_t size() const; size_t size_unprotected() const; void resize(size_t N); void reserve(size_t N); template <typename T> void setAllPoints( const std::vector<T>& x, const std::vector<T>& y, const std::vector<T>& z ); void setAllPoints(const std::vector<mrpt::math::TPoint3D>& pts); void setAllPointsFast(std::vector<mrpt::math::TPoint3Df>& pts); const std::vector<mrpt::math::TPoint3Df>& getArrayPoints() const; void clear(); bool empty() const; void insertPoint(float x, float y, float z); void insertPoint(const mrpt::math::TPoint3Df& p); void insertPoint(const mrpt::math::TPoint3D& p); const mrpt::math::TPoint3Df& operator [] (size_t i) const; const mrpt::math::TPoint3Df& getPoint3Df(size_t i) const; void setPoint(size_t i, const float x, const float y, const float z); void setPoint_fast(size_t i, const float x, const float y, const float z); template <class POINTSMAP> void loadFromPointsMap(const POINTSMAP* themap); template <class LISTOFPOINTS> void loadFromPointsList(LISTOFPOINTS& pointsList); size_t getActuallyRendered() const; void enableColorFromX(bool v = true); void enableColorFromY(bool v = true); void enableColorFromZ(bool v = true); void enablePointSmooth(bool enable = true); void disablePointSmooth(); bool isPointSmoothEnabled() const; void setGradientColors(const mrpt::img::TColorf& colorMin, const mrpt::img::TColorf& colorMax); virtual void onUpdateBuffers_Points(); void render_subset( const bool all, const std::vector<size_t>& idxs, const float render_area_sqpixels ) const; virtual void toYAMLMap(mrpt::containers::yaml& propertiesMap) const; };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; struct TNode; struct TRenderQueueElement; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Points() = 0; size_t octree_get_node_count() const; size_t octree_get_visible_nodes() const; void octree_mark_as_outdated(); void octree_get_graphics_boundingboxes( mrpt::opengl::CSetOfObjects& gl_bb, const float lines_width = 1, const mrpt::img::TColorf& lines_color = mrpt::img::TColorf(1, 1, 1), const bool draw_solid_boxes = false ) const; void octree_debug_dump_tree(std::ostream& o) const;
Construction
CPointCloud()
Constructor.
Methods
size_t size_unprotected() const
Like size(), but without locking the data mutex (internal usage)
void resize(size_t N)
Set the number of points (with contents undefined)
void reserve(size_t N)
Like STL std::vector’s reserve.
template <typename T> void setAllPoints( const std::vector<T>& x, const std::vector<T>& y, const std::vector<T>& z )
Set the list of (X,Y,Z) point coordinates, all at once, from three vectors with their coordinates.
void setAllPointsFast(std::vector<mrpt::math::TPoint3Df>& pts)
Set the list of (X,Y,Z) point coordinates, DESTROYING the contents of the input vectors (via swap)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
const std::vector<mrpt::math::TPoint3Df>& getArrayPoints() const
Get a const reference to the internal array of points.
void clear()
Empty the list of points.
void insertPoint(float x, float y, float z)
Adds a new point to the cloud.
const mrpt::math::TPoint3Df& operator [] (size_t i) const
Read access to each individual point (checks for “i” in the valid range only in Debug).
const mrpt::math::TPoint3Df& getPoint3Df(size_t i) const
NOTE: This method is intentionally not protected by the shared_mutex, since it’s called in the inner loops of the octree, which acquires the lock once.
void setPoint(size_t i, const float x, const float y, const float z)
Write an individual point (checks for “i” in the valid range only in Debug).
void setPoint_fast(size_t i, const float x, const float y, const float z)
Write an individual point (without checking validity of the index).
template <class POINTSMAP> void loadFromPointsMap(const POINTSMAP* themap)
Load the points from any other point map class supported by the adapter mrpt::opengl::PointCloudAdapter.
template <class LISTOFPOINTS> void loadFromPointsList(LISTOFPOINTS& pointsList)
Load the points from a list of mrpt::math::TPoint3D.
size_t getActuallyRendered() const
Get the number of elements actually rendered in the last render event.
void setGradientColors(const mrpt::img::TColorf& colorMin, const mrpt::img::TColorf& colorMax)
Sets the colors used as extremes when colorFromDepth is enabled.
virtual void onUpdateBuffers_Points()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
void render_subset( const bool all, const std::vector<size_t>& idxs, const float render_area_sqpixels ) const
Render a subset of points (required by octree renderer)
virtual void toYAMLMap(mrpt::containers::yaml& propertiesMap) const
Used from Scene::asYAML().
(New in MRPT 2.4.2)