class mrpt::opengl::CVectorField3D
Overview
A 3D vector field representation, consisting of points and arrows drawn at any spatial position.
This opengl object has been created to represent scene flow, and hence both the vector field and the coordinates of the points at which the vector field is represented are stored in matrices because they are computed from intensity and depth images.
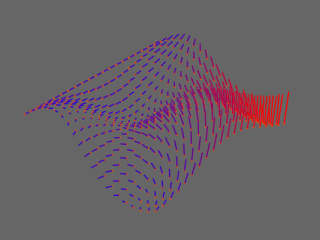
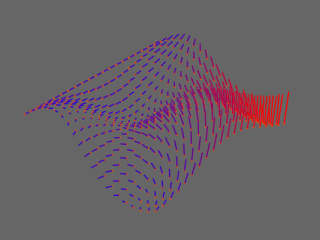
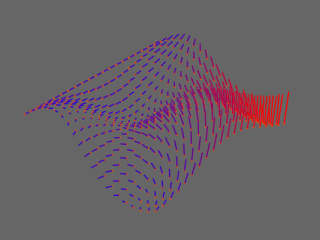
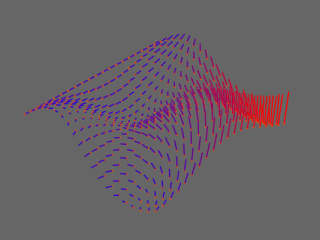
See also:
#include <mrpt/opengl/CVectorField3D.h> class CVectorField3D: public mrpt::opengl::CRenderizableShaderPoints, public mrpt::opengl::CRenderizableShaderWireFrame { public: // construction CVectorField3D(); CVectorField3D(mrpt::math::CMatrixFloat x_vf_ini, mrpt::math::CMatrixFloat y_vf_ini, mrpt::math::CMatrixFloat z_vf_ini, mrpt::math::CMatrixFloat x_p_ini, mrpt::math::CMatrixFloat y_p_ini, mrpt::math::CMatrixFloat z_p_ini); // methods virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual void freeOpenGLResources(); virtual shader_list_t requiredShaders() const; virtual void onUpdateBuffers_Wireframe(); virtual void onUpdateBuffers_Points(); const auto& shaderPointsVertexPointBuffer() const; const auto& shaderPointsVertexColorBuffer() const; auto& shaderPointsBuffersMutex() const; virtual CRenderizable& setColor_u8(const mrpt::img::TColor& c); const auto& shaderWireframeVertexPointBuffer() const; const auto& shaderWireframeVertexColorBuffer() const; auto& shaderWireframeBuffersMutex() const; void clear(); void setPointColor(const float R, const float G, const float B, const float A = 1); mrpt::img::TColorf getPointColor() const; void setVectorFieldColor(const float R, const float G, const float B, const float A = 1); void getVectorFieldColor(mrpt::img::TColorf Cmin, mrpt::img::TColorf Cmax) const; void setMotionFieldColormap( const float Rmin, const float Gmin, const float Bmin, const float Rmax, const float Gmax, const float Bmax, const float Amin = 1, const float Amax = 1 ); mrpt::img::TColorf getVectorFieldColor() const; void setMaxSpeedForColor(const float s); float getMaxSpeedForColor() const; void getVectorField(mrpt::math::CMatrixFloat& Matrix_x, mrpt::math::CMatrixFloat& Matrix_y, mrpt::math::CMatrixFloat& Matrix_z) const; template <class MATRIX> void getVectorField( MATRIX& Matrix_x, MATRIX& Matrix_y, MATRIX& Matrix_z ) const; void getPointCoordinates(mrpt::math::CMatrixFloat& Coord_x, mrpt::math::CMatrixFloat& Coord_y, mrpt::math::CMatrixFloat& Coord_z) const; template <class MATRIX> void getPointCoordinates( MATRIX& Coord_x, MATRIX& Coord_y, MATRIX& Coord_z ) const; const mrpt::math::CMatrixFloat& getVectorField_x() const; mrpt::math::CMatrixFloat& getVectorField_x(); const mrpt::math::CMatrixFloat& getVectorField_y() const; mrpt::math::CMatrixFloat& getVectorField_y(); const mrpt::math::CMatrixFloat& getVectorField_z() const; mrpt::math::CMatrixFloat& getVectorField_z(); void setVectorField(mrpt::math::CMatrixFloat& Matrix_x, mrpt::math::CMatrixFloat& Matrix_y, mrpt::math::CMatrixFloat& Matrix_z); template <class MATRIX> void setVectorField( MATRIX& Matrix_x, MATRIX& Matrix_y, MATRIX& Matrix_z ); void setPointCoordinates(mrpt::math::CMatrixFloat& Matrix_x, mrpt::math::CMatrixFloat& Matrix_y, mrpt::math::CMatrixFloat& Matrix_z); template <class MATRIX> void setPointCoordinates( MATRIX& Matrix_x, MATRIX& Matrix_y, MATRIX& Matrix_z ); void resize(size_t rows, size_t cols); size_t cols() const; size_t rows() const; virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; void enableColorFromModule(bool enable = true); void enableShowPoints(bool enable = true); bool isAntiAliasingEnabled() const; bool isColorFromModuleEnabled() const; float getPointSize() const; bool isEnabledVariablePointSize() const; float getVariablePointSize_k() const; float getVariablePointSize_DepthScale() const; void notifyBBoxChange() const; auto getBoundingBoxLocalf() const; void setLineWidth(float w); float getLineWidth() const; void enableAntiAliasing(bool enable = true); };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Points() = 0; virtual void onUpdateBuffers_Wireframe() = 0;
Construction
CVectorField3D()
Constructor.
CVectorField3D(mrpt::math::CMatrixFloat x_vf_ini, mrpt::math::CMatrixFloat y_vf_ini, mrpt::math::CMatrixFloat z_vf_ini, mrpt::math::CMatrixFloat x_p_ini, mrpt::math::CMatrixFloat y_p_ini, mrpt::math::CMatrixFloat z_p_ini)
Constructor with a initial set of lines.
Methods
virtual void render(const RenderContext& rc) const
Implements the rendering of 3D objects in each class derived from CRenderizable.
This can be called more than once (one per required shader program) if the object registered several shaders.
See also:
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual void freeOpenGLResources()
Free opengl buffers.
virtual shader_list_t requiredShaders() const
Returns the ID of the OpenGL shader program required to render this class.
See also:
virtual void onUpdateBuffers_Wireframe()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void onUpdateBuffers_Points()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
void clear()
Clear the matrices.
void setPointColor( const float R, const float G, const float B, const float A = 1 )
Set the point color in the range [0,1].
mrpt::img::TColorf getPointColor() const
Get the point color in the range [0,1].
void setVectorFieldColor( const float R, const float G, const float B, const float A = 1 )
Set the arrow color in the range [0,1].
void getVectorFieldColor(mrpt::img::TColorf Cmin, mrpt::img::TColorf Cmax) const
Get the motion field min and max colors (colormap) in the range [0,1].
void setMotionFieldColormap( const float Rmin, const float Gmin, const float Bmin, const float Rmax, const float Gmax, const float Bmax, const float Amin = 1, const float Amax = 1 )
Set the motion field min and max colors (colormap) in the range [0,1].
mrpt::img::TColorf getVectorFieldColor() const
Get the arrow color in the range [0,1].
void setMaxSpeedForColor(const float s)
Set the max speed associated for the color map ( m_still_color, m_maxspeed_color)
float getMaxSpeedForColor() const
Get the max_speed with which lines are drawn.
void getVectorField(mrpt::math::CMatrixFloat& Matrix_x, mrpt::math::CMatrixFloat& Matrix_y, mrpt::math::CMatrixFloat& Matrix_z) const
Get the vector field in three independent matrices: Matrix_x, Matrix_y and Matrix_z.
void getPointCoordinates(mrpt::math::CMatrixFloat& Coord_x, mrpt::math::CMatrixFloat& Coord_y, mrpt::math::CMatrixFloat& Coord_z) const
Get the coordiantes of the points at which the vector field is plotted: Coord_x, Coord_y and Coord_z.
const mrpt::math::CMatrixFloat& getVectorField_x() const
Get the “x” component of the vector field as a matrix.
mrpt::math::CMatrixFloat& getVectorField_x()
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
const mrpt::math::CMatrixFloat& getVectorField_y() const
Get the “y” component of the vector field as a matrix.
mrpt::math::CMatrixFloat& getVectorField_y()
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
const mrpt::math::CMatrixFloat& getVectorField_z() const
Get the “z” component of the vector field as a matrix.
mrpt::math::CMatrixFloat& getVectorField_z()
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void setVectorField(mrpt::math::CMatrixFloat& Matrix_x, mrpt::math::CMatrixFloat& Matrix_y, mrpt::math::CMatrixFloat& Matrix_z)
Set the vector field with Matrix_x, Matrix_y and Matrix_z.
void setPointCoordinates(mrpt::math::CMatrixFloat& Matrix_x, mrpt::math::CMatrixFloat& Matrix_y, mrpt::math::CMatrixFloat& Matrix_z)
Set the coordinates of the points at which the vector field is plotted with Matrix_x, Matrix_y and Matrix_z.
void resize(size_t rows, size_t cols)
Resizes the set.
size_t cols() const
Returns the total count of rows used to represent the vector field.
size_t rows() const
Returns the total count of columns used to represent the vector field.
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Must be implemented by derived classes to provide the updated bounding box in the object local frame of coordinates.
This will be called only once after each time the derived class reports to notifyChange() that the object geometry changed.
See also:
getBoundingBox(), getBoundingBoxLocal(), getBoundingBoxLocalf()