class mrpt::opengl::CMesh3D
Overview
A 3D mesh composed of triangles and/or quads.
A typical usage example would be a 3D model of an object.
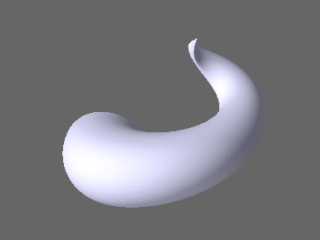
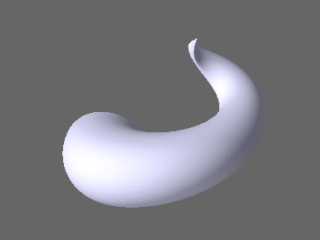
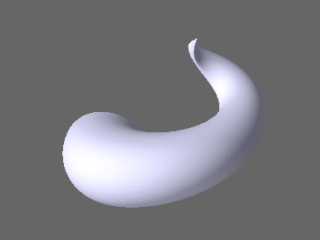
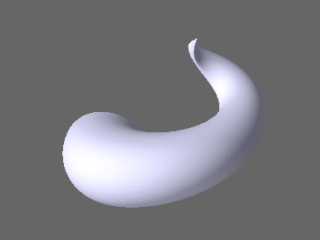
See also:
opengl::Scene, opengl::CMesh, opengl::CAssimpModel
#include <mrpt/opengl/CMesh3D.h> class CMesh3D: public mrpt::opengl::CRenderizableShaderTriangles, public mrpt::opengl::CRenderizableShaderWireFrame, public mrpt::opengl::CRenderizableShaderPoints { public: // construction CMesh3D(); // methods virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual void freeOpenGLResources(); virtual shader_list_t requiredShaders() const; virtual void onUpdateBuffers_Wireframe(); virtual void onUpdateBuffers_Triangles(); virtual void onUpdateBuffers_Points(); void enableShowEdges(bool v); void enableShowFaces(bool v); void enableShowVertices(bool v); void enableFaceNormals(bool v); void loadMesh( unsigned int num_verts, unsigned int num_faces, int* verts_per_face, int* face_verts, float* vert_coords ); void loadMesh( unsigned int num_verts, unsigned int num_faces, const mrpt::math::CMatrixDynamic<bool>& is_quad, const mrpt::math::CMatrixDynamic<int>& face_verts, const mrpt::math::CMatrixDynamic<float>& vert_coords ); void setEdgeColor( float r, float g, float b, float a = 1.f ); void setFaceColor( float r, float g, float b, float a = 1.f ); void setVertColor( float r, float g, float b, float a = 1.f ); virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Triangles() = 0; virtual void onUpdateBuffers_Wireframe() = 0; virtual void onUpdateBuffers_Points() = 0;
Methods
virtual void render(const RenderContext& rc) const
Implements the rendering of 3D objects in each class derived from CRenderizable.
This can be called more than once (one per required shader program) if the object registered several shaders.
See also:
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual void freeOpenGLResources()
Free opengl buffers.
virtual shader_list_t requiredShaders() const
Returns the ID of the OpenGL shader program required to render this class.
See also:
virtual void onUpdateBuffers_Wireframe()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void onUpdateBuffers_Triangles()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void onUpdateBuffers_Points()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
void loadMesh( unsigned int num_verts, unsigned int num_faces, int* verts_per_face, int* face_verts, float* vert_coords )
Load a 3D mesh.
The arguments indicate:
num_verts: Number of vertices of the mesh
num_faces: Number of faces of the mesh
verts_per_face: An array (pointer) with the number of vertices of each face. The elements must be set either to 3 (triangle) or 4 (quad).
face_verts: An array (pointer) with the vertices of each face. The vertices of each face must be consecutive in this array.
vert_coords: An array (pointer) with the coordinates of each vertex. The xyz coordinates of each vertex must be consecutive in this array.
void loadMesh( unsigned int num_verts, unsigned int num_faces, const mrpt::math::CMatrixDynamic<bool>& is_quad, const mrpt::math::CMatrixDynamic<int>& face_verts, const mrpt::math::CMatrixDynamic<float>& vert_coords )
Load a 3D mesh.
The arguments indicate:
num_verts: Number of vertices of the mesh
num_faces: Number of faces of the mesh
is_quad: A binary array saying whether the face is a quad (1) or a triangle (0)
face_verts: An array with the vertices of each face. For every column (face), each row contains the num of a vertex. The fourth does not need to be filled if the face is a triangle.
vert_coords: An array with the coordinates of each vertex. For every column (vertex), each row contains the xyz coordinates of the vertex.
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Must be implemented by derived classes to provide the updated bounding box in the object local frame of coordinates.
This will be called only once after each time the derived class reports to notifyChange() that the object geometry changed.
See also:
getBoundingBox(), getBoundingBoxLocal(), getBoundingBoxLocalf()