class mrpt::img::CImage
Overview
A class for storing images as grayscale or RGB bitmaps.
I/O is supported as:
Binary dump using the CSerializable interface(<< and >> operators), just as most objects in MRPT. This format is not compatible with any standarized image format but it is fast.
Saving/loading from files of different formats (bmp,jpg,png,…) using the methods CImage::loadFromFile and CImage::saveToFile. See OpenCV for the list of supported formats.
Importing from an XPM array (.xpm file format) using CImage::loadFromXPM()
Importing TGA images. See CImage::loadTGA()
How to create color/grayscale images:
CImage img1(width, height, CH_GRAY ); // Grayscale image (8U1C) CImage img2(width, height, CH_RGB ); // RGB image (8U3C)
Additional notes:
The OpenCV
cv::Mat
format is used internally for compatibility with all OpenCV functions. Use CImage::asCvMat() to retrieve it. Example:CImage img; ... cv::Mat m = img.asCvMat()
By default, all images use unsigned 8-bit storage format for pixels (on each channel), but it can be changed by flags in the constructor.
An external storage mode can be enabled by calling CImage::setExternalStorage, useful for storing large collections of image objects in memory while loading the image data itself only for the relevant images at any time. See CImage::forceLoad() and CImage::unload().
Operator = and copy ctor make shallow copies. For deep copies, see CImage::makeDeepCopy() or CImage(const CImage&, copy_type_t), e.g:
CImage a(20, 10, CH_GRAY); // Shallow copy ctor: CImage b(a, mrpt::img::SHALLOW_COPY); CImage c(a, mrpt::img::DEEP_COPY);
If you are interested in a smart pointer to an image, use:
CImage::Ptr myImg = CImage::Create(); // optional ctor arguments // or: CImage::Ptr myImg = std::make_shared<CImage>(...);
To set a CImage from an OpenCV
cv::Mat
use CImage::CImage(cv::Mat,copy_type_t).
Some functions are implemented in MRPT with highly optimized SSE2/SSE3 routines, in suitable platforms and compilers. To see the list of optimizations refer to sse_optimizations, falling back to default OpenCV methods where unavailable.
For computer vision functions that use CImage as its image data type, see mrpt::vision.
See also:
mrpt::vision, mrpt::vision::CFeatureExtractor, mrpt::vision::CImagePyramid, CSerializable, CCanvas
#include <mrpt/img/CImage.h> class CImage: public mrpt::serialization::CSerializable, public mrpt::img::CCanvas { public: // structs struct Impl; // construction CImage(); CImage(unsigned int width, unsigned int height, TImageChannels nChannels = CH_RGB); CImage(const CImage& other_img, ctor_CImage_ref_or_gray); CImage(const cv::Mat& img, copy_type_t copy_type); CImage(const CImage& img, copy_type_t copy_type); // methods void clear(); void resize(std::size_t width, std::size_t height, TImageChannels nChannels, PixelDepth depth = PixelDepth::D8U); PixelDepth getPixelDepth() const; void scaleImage(CImage& out_img, unsigned int width, unsigned int height, TInterpolationMethod interp = IMG_INTERP_CUBIC) const; void rotateImage( CImage& out_img, double ang, unsigned int cx, unsigned int cy, double scale = 1.0 ) const; virtual void setPixel(int x, int y, size_t color); virtual void line( int x0, int y0, int x1, int y1, const mrpt::img::TColor color, unsigned int width = 1, TPenStyle penStyle = psSolid ); virtual void drawCircle( int x, int y, int radius, const mrpt::img::TColor& color = mrpt::img::TColor(255, 255, 255), unsigned int width = 1 ); virtual void drawImage(int x, int y, const mrpt::img::CImage& img); virtual void filledRectangle(int x0, int y0, int x1, int y1, const mrpt::img::TColor color); void equalizeHist(CImage& out_img) const; CImage scaleHalf(TInterpolationMethod interp) const; bool scaleHalf(CImage& out_image, TInterpolationMethod interp) const; CImage scaleDouble(TInterpolationMethod interp) const; void scaleDouble(CImage& out_image, TInterpolationMethod interp) const; void update_patch(const CImage& patch, const unsigned int col, const unsigned int row); void extract_patch( CImage& patch, const unsigned int col = 0, const unsigned int row = 0, const unsigned int width = 1, const unsigned int height = 1 ) const; float correlate(const CImage& img2int, int width_init = 0, int height_init = 0) const; void cross_correlation_FFT( const CImage& in_img, math::CMatrixFloat& out_corr, int u_search_ini = -1, int v_search_ini = -1, int u_search_size = -1, int v_search_size = -1, float biasThisImg = 0, float biasInImg = 0 ) const; void normalize(); void flipVertical(); void flipHorizontal(); void swapRB(); void undistort(CImage& out_img, const mrpt::img::TCamera& cameraParams) const; void rectifyImageInPlace(void* mapX, void* mapY); void filterMedian(CImage& out_img, int W = 3) const; void filterGaussian(CImage& out_img, int W = 3, int H = 3, double sigma = 1.0) const; bool drawChessboardCorners( const std::vector<TPixelCoordf>& cornerCoords, unsigned int check_size_x, unsigned int check_size_y, unsigned int lines_width = 1, unsigned int circles_radius = 4 ); void joinImagesHorz(const CImage& im1, const CImage& im2); float KLT_response( const unsigned int x, const unsigned int y, const unsigned int half_window_size ) const; CImage makeShallowCopy() const; CImage makeDeepCopy() const; void copyFromForceLoad(const CImage& o); void loadFromIplImage(const IplImage* iplImage, copy_type_t c = DEEP_COPY); void swap(CImage& o); void asCvMat(cv::Mat& out_img, copy_type_t copy_type) const; template <typename CV_MAT> CV_MAT asCvMat(copy_type_t copy_type) const; cv::Mat& asCvMatRef(); const cv::Mat& asCvMatRef() const; template <typename T> const T& at( unsigned int col, unsigned int row, unsigned int channel = 0 ) const; template <typename T> T& at( unsigned int col, unsigned int row, unsigned int channel = 0 ); template <typename T> const T* ptr( unsigned int col, unsigned int row, unsigned int channel = 0 ) const; template <typename T> T* ptr( unsigned int col, unsigned int row, unsigned int channel = 0 ); template <typename T> const T* ptrLine(unsigned int row) const; template <typename T> T* ptrLine(unsigned int row); float getAsFloat(unsigned int col, unsigned int row, unsigned int channel) const; float getAsFloat(unsigned int col, unsigned int row) const; unsigned char* operator () (unsigned int col, unsigned int row, unsigned int channel = 0) const; virtual size_t getWidth() const; virtual size_t getHeight() const; void getSize(TImageSize& s) const; TImageSize getSize() const; size_t getRowStride() const; std::string getChannelsOrder() const; float getMaxAsFloat() const; int channelCount() const; bool isColor() const; bool isEmpty() const; bool isOriginTopLeft() const; TImageChannels getChannelCount() const; void getAsMatrix( mrpt::math::CMatrixFloat& outMatrix, bool doResize = true, int x_min = 0, int y_min = 0, int x_max = -1, int y_max = -1, bool normalize_01 = true ) const; void getAsMatrix( mrpt::math::CMatrix_u8& outMatrix, bool doResize = true, int x_min = 0, int y_min = 0, int x_max = -1, int y_max = -1 ) const; void getAsRGBMatrices( mrpt::math::CMatrixFloat& outMatrixR, mrpt::math::CMatrixFloat& outMatrixG, mrpt::math::CMatrixFloat& outMatrixB, bool doResize = true, int x_min = 0, int y_min = 0, int x_max = -1, int y_max = -1 ) const; void getAsRGBMatrices( mrpt::math::CMatrix_u8& outMatrixR, mrpt::math::CMatrix_u8& outMatrixG, mrpt::math::CMatrix_u8& outMatrixB, bool doResize = true, int x_min = 0, int y_min = 0, int x_max = -1, int y_max = -1 ) const; void getAsMatrixTiled(mrpt::math::CMatrixFloat& outMatrix) const; void setExternalStorage(const std::string& fileName); bool isExternallyStored() const; std::string getExternalStorageFile() const; void getExternalStorageFileAbsolutePath(std::string& out_path) const; std::string getExternalStorageFileAbsolutePath() const; void forceLoad() const; void unload() const; static const std::string& getImagesPathBase(); static void setImagesPathBase(const std::string& path); void loadFromMemoryBuffer( unsigned int width, unsigned int height, bool color, unsigned char* rawpixels, bool swapRedBlue = false ); void loadFromMemoryBuffer( unsigned int width, unsigned int height, unsigned int bytesPerRow, unsigned char* red, unsigned char* green, unsigned char* blue ); template <typename MAT> void setFromMatrix( const MAT& m, bool matrix_is_normalized = true, bool flip_vertically = false ); template <typename MAT> void setFromRGBMatrices( const MAT& r, const MAT& g, const MAT& b, bool matrix_is_normalized = true ); void loadFromStreamAsJPEG(mrpt::io::CStream& in); bool loadFromFile(const std::string& fileName, int isColor = -1); bool loadFromXPM(const char*const* xpm_array, bool swap_rb = true); bool saveToFile(const std::string& fileName, int jpeg_quality = 95) const; void saveToStreamAsJPEG(mrpt::io::CStream& out, const int jpeg_quality = 95) const; static mrpt::img::CImage LoadFromFile(const std::string& fileName, int isColor = -1); static bool loadTGA( const std::string& fileName, mrpt::img::CImage& out_RGB, mrpt::img::CImage& out_alpha ); CImage grayscale() const; bool grayscale(CImage& ret) const; CImage colorImage() const; void colorImage(CImage& ret) const; static void DISABLE_JPEG_COMPRESSION(bool val); static bool DISABLE_JPEG_COMPRESSION(); static void SERIALIZATION_JPEG_QUALITY(int q); static int SERIALIZATION_JPEG_QUALITY(); void getAsIplImage(IplImage* dest) const; };
Inherited Members
public: // enums enum TPenStyle; // methods virtual void setPixel(int x, int y, size_t color) = 0; virtual size_t getWidth() const = 0; virtual size_t getHeight() const = 0; virtual void line( int x0, int y0, int x1, int y1, const mrpt::img::TColor color, unsigned int width = 1, TPenStyle penStyle = psSolid ); void rectangle(int x0, int y0, int x1, int y1, const mrpt::img::TColor color, unsigned int width = 1); void triangle( int x0, int y0, int size, const mrpt::img::TColor color, bool inferior = true, unsigned int width = 1 ); virtual void filledRectangle(int x0, int y0, int x1, int y1, const mrpt::img::TColor color); virtual void textOut(int x0, int y0, const std::string& str, const mrpt::img::TColor color); virtual void selectTextFont(const std::string& fontName); virtual void drawImage(int x, int y, const mrpt::img::CImage& img); void drawMark( int x0, int y0, const mrpt::img::TColor color, char type, int size = 5, unsigned int width = 1 ); virtual void drawImage( int x, int y, const mrpt::img::CImage& img, float rotation, float scale ); virtual void drawCircle( int x, int y, int radius, const mrpt::img::TColor& color = mrpt::img::TColor(255, 255, 255), unsigned int width = 1 ); void ellipseGaussian( const mrpt::math::CMatrixFixed<double, 2, 2>& cov2D, const double mean_x, const double mean_y, double confIntervalStds = 2, const mrpt::img::TColor& color = mrpt::img::TColor(255, 255, 255), unsigned int width = 1, int nEllipsePoints = 20 ); template <class FEATURELIST> void drawFeaturesSimple( const FEATURELIST& list, const TColor& color = TColor::red(), const int cross_size = 5 ); template <class FEATURELIST> void drawFeatures( const FEATURELIST& list, const TColor& color = TColor::red(), const bool showIDs = false, const bool showResponse = false, const bool showScale = false, const char marker = '+' );
Construction
CImage()
Default constructor: initialize to empty image.
It’s an error trying to access the image in such a state (except reading the image width/height, which are both zero). Either call resize(), assign from another image, load from disk, deserialize from an archive, etc. to properly initialize the image.
CImage(unsigned int width, unsigned int height, TImageChannels nChannels = CH_RGB)
Constructor for a given image size and type.
Examples:
CImage img1(width, height, CH_GRAY ); // Grayscale image (8U1C) CImage img2(width, height, CH_RGB ); // RGB image (8U3C)
CImage(const CImage& other_img, ctor_CImage_ref_or_gray)
Fast constructor of a grayscale version of another image, making a shallow copy from the original image if it already was grayscale, or otherwise creating a new grayscale image and converting the original image into it.
Example of usage:
void my_func(const CImage &in_img) { const CImage gray_img(in_img, FAST_REF_OR_CONVERT_TO_GRAY); // We can now operate on "gray_img" being sure it's in grayscale. }
CImage(const cv::Mat& img, copy_type_t copy_type)
Constructor from a cv::Mat image, making or not a deep copy of the data.
CImage(const CImage& img, copy_type_t copy_type)
Constructor from another CImage, making or not a deep copy of the data.
Methods
void clear()
Resets the image to the state after a default ctor.
Accessing the image after will throw an exception, unless it is formerly initialized somehow: loading an image from disk, calling rezize(), etc.
void resize(std::size_t width, std::size_t height, TImageChannels nChannels, PixelDepth depth = PixelDepth::D8U)
Changes the size of the image, erasing previous contents (does NOT scale its current content, for that, see scaleImage).
nChannels: Can be 3 for RGB images or 1 for grayscale images.
See also:
void scaleImage( CImage& out_img, unsigned int width, unsigned int height, TInterpolationMethod interp = IMG_INTERP_CUBIC ) const
Scales this image to a new size, interpolating as needed, saving the new image in a different output object, or operating in-place if out_img==this
.
See also:
void rotateImage( CImage& out_img, double ang, unsigned int cx, unsigned int cy, double scale = 1.0 ) const
Rotates the image by the given angle (in radians) around the given center point, with an optional scale factor.
The output image will have the same size as the input, except if angle is exactly ±90 degrees, in which case a quick image rotation (switching height and widht) will be performed instead.
See also:
virtual void setPixel(int x, int y, size_t color)
Changes the value of the pixel (x,y).
Pixel coordinates starts at the left-top corner of the image, and start in (0,0). The meaning of the parameter “color” depends on the implementation: it will usually be a 24bit RGB value (0x00RRGGBB), but it can also be just a 8bit gray level. This method must support (x,y) values OUT of the actual image size without neither raising exceptions, nor leading to memory access errors.
See also:
virtual void line( int x0, int y0, int x1, int y1, const mrpt::img::TColor color, unsigned int width = 1, TPenStyle penStyle = psSolid )
Draws a line.
Parameters:
x0 |
The starting point x coordinate |
y0 |
The starting point y coordinate |
x1 |
The end point x coordinate |
y1 |
The end point y coordinate |
color |
The color of the line |
width |
The desired width of the line (this is IGNORED in this virtual class) This method may be redefined in some classes implementing this interface in a more appropriate manner. |
virtual void drawCircle( int x, int y, int radius, const mrpt::img::TColor& color = mrpt::img::TColor(255, 255, 255), unsigned int width = 1 )
Draws a circle of a given radius.
Parameters:
x |
The center - x coordinate in pixels. |
y |
The center - y coordinate in pixels. |
radius |
The radius - in pixels. |
color |
The color of the circle. |
width |
The desired width of the line (this is IGNORED in this virtual class) |
virtual void drawImage(int x, int y, const mrpt::img::CImage& img)
Draws an image as a bitmap at a given position.
Parameters:
x0 |
The top-left corner x coordinates on this canvas where the image is to be drawn |
y0 |
The top-left corner y coordinates on this canvas where the image is to be drawn |
img |
The image to be drawn in this canvas This method may be redefined in some classes implementing this interface in a more appropriate manner. |
virtual void filledRectangle(int x0, int y0, int x1, int y1, const mrpt::img::TColor color)
Draws a filled rectangle.
Parameters:
x0 |
The top-left x coordinate |
y0 |
The top-left y coordinate |
x1 |
The right-bottom x coordinate |
y1 |
The right-bottom y coordinate |
color |
The color of the rectangle fill This method may be redefined in some classes implementing this interface in a more appropriate manner. |
See also:
void equalizeHist(CImage& out_img) const
Equalize the image histogram, saving the new image in the given output object.
RGB images are first converted to HSV color space, then equalized for brightness (V)
CImage scaleHalf(TInterpolationMethod interp) const
Returns a new image scaled down to half its original size.
Parameters:
std::exception |
On odd size |
See also:
scaleDouble, scaleImage, scaleHalfSmooth
bool scaleHalf(CImage& out_image, TInterpolationMethod interp) const
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Returns:
true if an optimized SSE2/SSE3 version could be used.
CImage scaleDouble(TInterpolationMethod interp) const
Returns a new image scaled up to double its original size.
Parameters:
std::exception |
On odd size |
See also:
void scaleDouble(CImage& out_image, TInterpolationMethod interp) const
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void update_patch( const CImage& patch, const unsigned int col, const unsigned int row )
Update a part of this image with the “patch” given as argument.
The “patch” will be “pasted” at the (col,row) coordinates of this image.
Parameters:
std::exception |
if patch pasted on the pixel (_row, _column) jut out of the image. |
See also:
void extract_patch( CImage& patch, const unsigned int col = 0, const unsigned int row = 0, const unsigned int width = 1, const unsigned int height = 1 ) const
Extract a patch from this image, saveing it into “patch” (its previous contents will be overwritten).
The patch to extract starts at (col,row) and has the given dimensions.
See also:
float correlate(const CImage& img2int, int width_init = 0, int height_init = 0) const
Computes the correlation coefficient (returned as val), between two images This function use grayscale images only img1, img2 must be same size (by AJOGD @ DEC-2006)
void cross_correlation_FFT( const CImage& in_img, math::CMatrixFloat& out_corr, int u_search_ini = -1, int v_search_ini = -1, int u_search_size = -1, int v_search_size = -1, float biasThisImg = 0, float biasInImg = 0 ) const
Computes the correlation matrix between this image and another one.
This implementation uses the 2D FFT for achieving reduced computation time.
Parameters:
in_img |
The “patch” image, which must be equal, or smaller than “this” image. This function supports gray-scale (1 channel only) images. |
u_search_ini |
The “x” coordinate of the search window. |
v_search_ini |
The “y” coordinate of the search window. |
u_search_size |
The width of the search window. |
v_search_size |
The height of the search window. |
out_corr |
The output for the correlation matrix, which will be “u_search_size” x “v_search_size” |
biasThisImg |
This optional parameter is a fixed “bias” value to be subtracted to the pixels of “this” image before performing correlation. |
biasInImg |
This optional parameter is a fixed “bias” value to be subtracted to the pixels of “in_img” image before performing correlation. Note: By default, the search area is the whole (this) image. (by JLBC @ JAN-2006) |
See also:
cross_correlation
void normalize()
Optimize the brightness range of an image without using histogram Only for one channel images.
See also:
void flipVertical()
Flips the image vertically.
See also:
void flipHorizontal()
Flips the image horizontally.
See also:
void swapRB()
Swaps red and blue channels.
void undistort(CImage& out_img, const mrpt::img::TCamera& cameraParams) const
Undistort the image according to some camera parameters, and returns an output undistorted image.
The intrinsic parameters (fx,fy,cx,cy) of the output image are the same than in the input image.
Parameters:
out_img |
The output undistorted image |
cameraParams |
The input camera params (containing the intrinsic and distortion parameters of the camera) |
See also:
void rectifyImageInPlace(void* mapX, void* mapY)
Rectify an image (undistorts and rectification) from a stereo pair according to a pair of precomputed rectification maps.
Parameters:
mapX |
|
mapY |
[IN] The pre-computed maps of the rectification (should be computed beforehand) |
See also:
mrpt::vision::CStereoRectifyMap, mrpt::vision::computeStereoRectificationMaps
void filterMedian(CImage& out_img, int W = 3) const
Filter the image with a Median filter with a window size WxW, returning the filtered image in out_img.
For inplace operation, set out_img to this.
void filterGaussian(CImage& out_img, int W = 3, int H = 3, double sigma = 1.0) const
Filter the image with a Gaussian filter with a window size WxH, replacing “this” image by the filtered one.
For inplace operation, set out_img to this.
bool drawChessboardCorners( const std::vector<TPixelCoordf>& cornerCoords, unsigned int check_size_x, unsigned int check_size_y, unsigned int lines_width = 1, unsigned int circles_radius = 4 )
Draw onto this image the detected corners of a chessboard.
The length of cornerCoords must be the product of the two check_sizes.
Parameters:
cornerCoords |
[IN] The pixel coordinates of all the corners. |
check_size_x |
[IN] The number of squares, in the X direction |
check_size_y |
[IN] The number of squares, in the Y direction |
Returns:
false if the length of cornerCoords is inconsistent (nothing is drawn then).
See also:
mrpt::vision::findChessboardCorners
void joinImagesHorz(const CImage& im1, const CImage& im2)
Joins two images side-by-side horizontally.
Both images must have the same number of rows and be of the same type (i.e. depth and color mode)
Parameters:
im1 |
[IN] The first image. |
im2 |
[IN] The other image. |
float KLT_response( const unsigned int x, const unsigned int y, const unsigned int half_window_size ) const
Compute the KLT response at a given pixel (x,y) - Only for grayscale images (for efficiency it avoids converting to grayscale internally).
See KLT_response() for more details on the internal optimizations of this method, but this graph shows a general view:
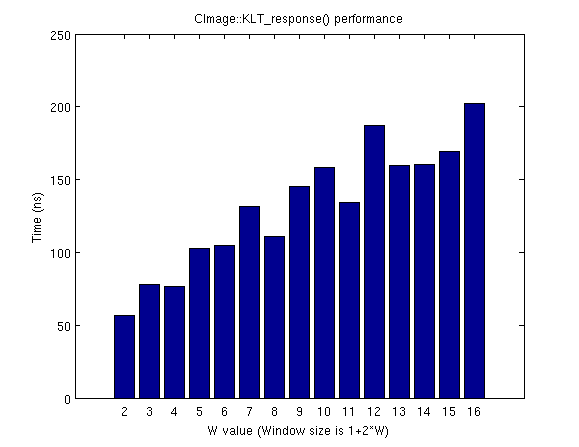
CImage makeShallowCopy() const
Returns a shallow copy of the original image.
CImage makeDeepCopy() const
Returns a deep copy of this image.
If the image is externally-stored, there is no difference with a shallow copy.
See also:
void copyFromForceLoad(const CImage& o)
Copies from another image (shallow copy), and, if it is externally stored, the image file will be actually loaded into memory in “this” object.
Parameters:
If the external image couldn’t be loaded. |
See also:
operator =
void loadFromIplImage(const IplImage* iplImage, copy_type_t c = DEEP_COPY)
Moves an image from another object, erasing the origin image in the process.
See also:
operator = Assigns from an image in IplImage format
void swap(CImage& o)
Efficiently swap of two images.
void asCvMat(cv::Mat& out_img, copy_type_t copy_type) const
Makes a shallow or deep copy of this image into the provided cv::Mat.
See also:
cv::Mat& asCvMatRef()
Get a reference to the internal cv::Mat, which can be resized, etc.
and changes will be reflected in this CImage object.
template <typename T> const T& at( unsigned int col, unsigned int row, unsigned int channel = 0 ) const
Access to pixels without checking boundaries - Use normally the () operator better, which checks the coordinates.
See also:
CImage::operator() Access to pixels without checking boundaries, and doing a reinterpret_cast<> of the data as the given type.
The CImage::operator() which does check for coordinate limits.
template <typename T> const T* ptr( unsigned int col, unsigned int row, unsigned int channel = 0 ) const
Returns a pointer to a given pixel, without checking for boundaries.
See also:
The CImage::operator() which does check for coordinate limits.
template <typename T> const T* ptrLine(unsigned int row) const
Returns a pointer to the first pixel of the given line.
See also:
float getAsFloat(unsigned int col, unsigned int row, unsigned int channel) const
Returns the contents of a given pixel at the desired channel, in float format: [0,255]->[0,1] The coordinate origin is pixel(0,0)=top-left corner of the image.
Parameters:
std::exception |
On pixel coordinates out of bounds |
See also:
operator()
float getAsFloat(unsigned int col, unsigned int row) const
Returns the contents of a given pixel (for gray-scale images, in color images the gray scale equivalent is computed for the pixel), in float format: [0,255]->[0,1] The coordinate origin is pixel(0,0)=top-left corner of the image.
Parameters:
std::exception |
On pixel coordinates out of bounds |
See also:
operator()
unsigned char* operator () ( unsigned int col, unsigned int row, unsigned int channel = 0 ) const
Returns a pointer to a given pixel information.
The coordinate origin is pixel(0,0)=top-left corner of the image.
Parameters:
std::exception |
On pixel coordinates out of bounds |
virtual size_t getWidth() const
Returns the width of the image in pixels.
See also:
virtual size_t getHeight() const
Returns the height of the image in pixels.
See also:
void getSize(TImageSize& s) const
Return the size of the image.
See also:
TImageSize getSize() const
Return the size of the image.
See also:
size_t getRowStride() const
Returns the row stride of the image: this is the number of bytes between two consecutive rows.
You can access the pointer to the first row with ptrLine(0)
See also:
std::string getChannelsOrder() const
As of mrpt 2.0.0, this returns either “GRAY” or “BGR”.
float getMaxAsFloat() const
Return the maximum pixel value of the image, as a float value in the range [0,1].
See also:
int channelCount() const
Returns 1 (grayscale), 3 (RGB) or 4 (RGBA) [New in MRPT 2.1.1].
bool isColor() const
Returns true if the image is RGB or RGBA, false if it is grayscale.
bool isEmpty() const
Returns true if the object is in the state after default constructor.
Returns false for delay-loaded images, disregarding whether the image is actually on disk or memory.
bool isOriginTopLeft() const
Returns true (as of MRPT v2.0.0, it’s fixed)
TImageChannels getChannelCount() const
Returns the number of channels, typically 1 (GRAY) or 3 (RGB)
See also:
void getAsMatrix( mrpt::math::CMatrixFloat& outMatrix, bool doResize = true, int x_min = 0, int y_min = 0, int x_max = -1, int y_max = -1, bool normalize_01 = true ) const
Returns the image as a matrix with pixel grayscale values in the range [0,1].
Matrix indexes in this order: M(row,column)
Parameters:
doResize |
If set to true (default), the output matrix will be always the size of the image at output. If set to false, the matrix will be enlarged to the size of the image, but it will not be cropped if it has room enough (useful for FFT2D,…) |
x_min |
The starting “x” coordinate to extract (default=0=the first column) |
y_min |
The starting “y” coordinate to extract (default=0=the first row) |
x_max |
The final “x” coordinate (inclusive) to extract (default=-1=the last column) |
y_max |
The final “y” coordinate (inclusive) to extract (default=-1=the last row) |
normalize_01 |
Normalize the image values such that they fall in the range [0,1] (default: true). If set to false, the matrix will hold numbers in the range [0,255]. |
See also:
void getAsRGBMatrices( mrpt::math::CMatrixFloat& outMatrixR, mrpt::math::CMatrixFloat& outMatrixG, mrpt::math::CMatrixFloat& outMatrixB, bool doResize = true, int x_min = 0, int y_min = 0, int x_max = -1, int y_max = -1 ) const
Returns the image as RGB matrices with pixel values in the range [0,1].
Matrix indexes in this order: M(row,column)
Parameters:
doResize |
If set to true (default), the output matrix will be always the size of the image at output. If set to false, the matrix will be enlarged to the size of the image, but it will not be cropped if it has room enough (useful for FFT2D,…) |
x_min |
The starting “x” coordinate to extract (default=0=the first column) |
y_min |
The starting “y” coordinate to extract (default=0=the first row) |
x_max |
The final “x” coordinate (inclusive) to extract (default=-1=the last column) |
y_max |
The final “y” coordinate (inclusive) to extract (default=-1=the last row) |
See also:
void getAsMatrixTiled(mrpt::math::CMatrixFloat& outMatrix) const
Returns the image as a matrix, where the image is “tiled” (repeated) the required number of times to fill the entire size of the matrix on input.
void setExternalStorage(const std::string& fileName)
By using this method the image is marked as referenced to an external file, which will be loaded only under demand.
A CImage with external storage does not consume memory until some method trying to access the image is invoked (e.g. getWidth(), isColor(),…) At any moment, the image can be unloaded from memory again by invoking unload. An image becomes of type “external storage” only through calling setExternalStorage. This property remains after serializing the object. File names can be absolute, or relative to the CImage::getImagesPathBase() directory. Filenames staring with “X:" or “/” are considered absolute paths. By calling this method the current contents of the image are NOT saved to that file, because this method can be also called to let the object know where to load the image in case its contents are required. Thus, for saving images in this format (not when loading) the proper order of commands should be:
img.saveToFile( fileName ); img.setExternalStorage( fileName );
Modifications to the memory copy of the image are not automatically saved to disk.
See also:
bool isExternallyStored() const
See setExternalStorage().
std::string getExternalStorageFile() const
Only if isExternallyStored() returns true.
See also:
getExternalStorageFileAbsolutePath
void getExternalStorageFileAbsolutePath(std::string& out_path) const
Only if isExternallyStored() returns true.
See also:
std::string getExternalStorageFileAbsolutePath() const
Only if isExternallyStored() returns true.
See also:
void forceLoad() const
For external storage image objects only, this method makes sure the image is loaded in memory.
Note that usually images are loaded on-the-fly on first access and there’s no need to call this. unload
void unload() const
For external storage image objects only, this method unloads the image from memory (or does nothing if already unloaded).
It does not need to be called explicitly, unless the user wants to save memory for images that will not be used often. If called for an image without the flag “external storage”, it is simply ignored.
See also:
static const std::string& getImagesPathBase()
By default, “.”.
Since MRPT 2.3.3 this is a synonym with mrpt::io::getLazyLoadPathBase()
See also:
static void setImagesPathBase(const std::string& path)
Since MRPT 2.3.3 this is a synonym with mrpt::io::setLazyLoadPathBase()
void loadFromMemoryBuffer( unsigned int width, unsigned int height, bool color, unsigned char* rawpixels, bool swapRedBlue = false )
Reads the image from raw pixels buffer in memory.
void loadFromMemoryBuffer( unsigned int width, unsigned int height, unsigned int bytesPerRow, unsigned char* red, unsigned char* green, unsigned char* blue )
Reads a color image from three raw pixels buffers in memory.
bytesPerRow is the number of bytes per row per channel, i.e. the row increment.
template <typename MAT> void setFromMatrix( const MAT& m, bool matrix_is_normalized = true, bool flip_vertically = false )
Set the image from a matrix, interpreted as grayscale intensity values, in the range [0,1] (normalized=true) or [0,255] (normalized=false) Matrix indexes are assumed to be in this order: M(row,column)
See also:
template <typename MAT> void setFromRGBMatrices( const MAT& r, const MAT& g, const MAT& b, bool matrix_is_normalized = true )
Set the image from RGB matrices, given the pixels in the range [0,1] (normalized=true) or [0,255] (normalized=false) Matrix indexes are assumed to be in this order: M(row,column)
See also:
void loadFromStreamAsJPEG(mrpt::io::CStream& in)
Reads the image from a binary stream containing a binary jpeg file.
Parameters:
std::exception |
On pixel coordinates out of bounds |
bool loadFromFile(const std::string& fileName, int isColor = -1)
Load image from a file, whose format is determined from the extension (internally uses OpenCV).
Windows bitmaps - BMP, DIB;
JPEG files - JPEG, JPG, JPE;
Portable Network Graphics - PNG;
Portable image format - PBM, PGM, PPM;
Sun rasters - SR, RAS;
TIFF files - TIFF, TIF.
MRPT also provides the special loaders loadFromXPM() and loadTGA().
Note that this function uses cv::imdecode() internally to reuse the memory buffer used by the image already loaded into this CImage, if possible, minimizing the number of memory allocations.
Parameters:
fileName |
The file to read from. |
isColor |
Specifies colorness of the loaded image:
|
Returns:
False on any error
See also:
saveToFile, setExternalStorage, loadFromXPM, loadTGA
bool loadFromXPM(const char*const* xpm_array, bool swap_rb = true)
Loads the image from an XPM array, as included from a “.xpm” file.
Parameters:
swap_rb |
Swaps red/blue channels from loaded image. Seems to be always needed, so it’s enabled by default. |
Returns:
false on any error
false on any error
See also:
bool saveToFile(const std::string& fileName, int jpeg_quality = 95) const
Save the image to a file, whose format is determined from the extension (internally uses OpenCV).
The supported formats are:
Windows bitmaps - BMP, DIB;
JPEG files - JPEG, JPG, JPE;
Portable Network Graphics - PNG;
Portable image format - PBM, PGM, PPM;
Sun rasters - SR, RAS;
TIFF files - TIFF, TIF.
jpeg_quality is only effective if MRPT is compiled against OpenCV 1.1.0 or newer.
Parameters:
fileName |
The file to write to. |
jpeg_quality |
Only for JPEG files, the quality of the compression in the range [0-100]. Larger is better quality but slower. |
Returns:
False on any error
See also:
void saveToStreamAsJPEG(mrpt::io::CStream& out, const int jpeg_quality = 95) const
Save image to binary stream as a JPEG (.jpg) compressed format.
Parameters:
std::exception |
On number of rows or cols equal to zero or other errors. |
See also:
saveToJPEG
static mrpt::img::CImage LoadFromFile( const std::string& fileName, int isColor = -1 )
Static method to construct an CImage object from a file.
See CImage::loadFromFile() for meaning of parameters.
New in MRPT 2.4.2
Parameters:
std::exception |
On load error. |
static bool loadTGA( const std::string& fileName, mrpt::img::CImage& out_RGB, mrpt::img::CImage& out_alpha )
Loads a TGA true-color RGBA image as two CImage objects, one for the RGB channels plus a separate gray-level image with A channel.
Returns:
true on success
CImage grayscale() const
Returns a grayscale version of the image, or a shallow copy of itself if it is already a grayscale image.
CImage colorImage() const
Returns a color (RGB) version of the grayscale image, or a shallow copy of itself if it is already a color image.
See also:
static void DISABLE_JPEG_COMPRESSION(bool val)
By default, when storing images through the CSerializable interface, RGB images are JPEG-compressed to save space.
If for some reason you prefer storing RAW image data, disable this feature by setting this flag to true. (Default = true)
static void SERIALIZATION_JPEG_QUALITY(int q)
Unless DISABLE_JPEG_COMPRESSION=true, this sets the JPEG quality (range 1-100) of serialized RGB images.
(Default = 95)
void getAsIplImage(IplImage* dest) const
(DEPRECATED, DO NOT USE - Kept here only to interface opencv 2.4)