Python example: opengl-demo-gui.py
This example illustrates how to create a 3D GUI in MRPT using the Python API and populate it with different objects, including animating them across the scene.
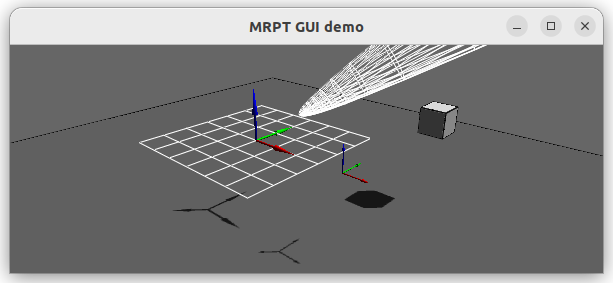
1#!/usr/bin/env python3
2
3# ---------------------------------------------------------------------
4# Install python3-pymrpt, ros-$ROS_DISTRO-python-mrpt,
5# ros-$ROS_DISTRO-mrpt2, or test with a local build with:
6# export PYTHONPATH=$HOME/code/mrpt/build-Release/:$PYTHONPATH
7# ---------------------------------------------------------------------
8
9from mrpt.pymrpt import mrpt
10import time
11import math
12
13# Create GUI:
14win = mrpt.gui.CDisplayWindow3D('MRPT GUI demo', 800, 600)
15
16# Get and lock 3D scene:
17# Lock is required again each time the scene is modified, to prevent
18# data race between the main and the rendering threads.
19scene = win.get3DSceneAndLock()
20
21# A grid on the XY horizontal plane:
22# ctor args: xMin: float, xMax: float, yMin: float, yMax: float, z: float, frequency: float
23glGrid = mrpt.opengl.CGridPlaneXY.Create(-3, 3, -3, 3, 0, 1)
24scene.insert(glGrid)
25
26# A couple of XYZ "corners":
27glCorner = mrpt.opengl.stock_objects.CornerXYZ(2.0)
28scene.insert(glCorner)
29
30glCorner2: mrpt.opengl.CSetOfObjects = mrpt.opengl.stock_objects.CornerXYZ(1.0)
31glCorner2.setLocation(4.0, 0.0, 0.0)
32scene.insert(glCorner2)
33
34# A 3D inverse-depth ellipsoid:
35glEllip = mrpt.opengl.CEllipsoidInverseDepth3D()
36cov = mrpt.math.CMatrixFixed_double_3UL_3UL_t.Zero()
37cov[0, 0] = 0.01
38cov[1, 1] = 0.001
39cov[2, 2] = 0.002
40mean = mrpt.math.CMatrixFixed_double_3UL_1UL_t()
41mean[0, 0] = 0.2 # inv_range
42mean[1, 0] = 0.5 # yaw
43mean[2, 0] = -0.6 # pitch
44glEllip.setCovMatrixAndMean(cov, mean)
45scene.insert(glEllip)
46
47# A floor "block":
48glFloor = mrpt.opengl.CBox()
49glFloor.setBoxCorners(mrpt.math.TPoint3D_double_t(-15, -15, 0),
50 mrpt.math.TPoint3D_double_t(15, 15, 0.1))
51glFloor.setLocation(0, 0, -3.0)
52glFloor.setColor_u8(mrpt.img.TColor(0x70, 0x70, 0x70))
53scene.insert(glFloor)
54
55# A mobile box to illustrate animations:
56glBox = mrpt.opengl.CBox()
57glBox.setBoxCorners(mrpt.math.TPoint3D_double_t(0, 0, 0),
58 mrpt.math.TPoint3D_double_t(1, 1, 1))
59glBox.setBoxBorderColor(mrpt.img.TColor(0, 0, 0))
60glBox.castShadows(True)
61scene.insert(glBox)
62
63# Shadows are disabled by default, enable them:
64scene.getViewport().enableShadowCasting(True)
65print('Shadow casting: ' + str(scene.getViewport().isShadowCastingEnabled()))
66
67# Move camera:
68win.setCameraAzimuthDeg(-40.0)
69win.setCameraElevationDeg(30.0)
70
71# end of scene lock:
72win.unlockAccess3DScene()
73
74
75print('Close the window to quit the program')
76timer = mrpt.system.CTicTac()
77
78while win.isOpen():
79 y = 5.0*math.sin(1.0*timer.Tac())
80 glBox.setLocation(4.0, y, 0.0)
81
82 win.repaint()
83 time.sleep(50e-3)