class mrpt::opengl::CText3D
A 3D text (rendered with OpenGL primitives), with selectable font face and drawing style.
Use setString and setFont to change the text displayed by this object (can be multi-lined).
Text is drawn along the (+X,+Y) axes.
Default size of characters is “1.0 units”. Change it with the standard method CRenderizable::setScale() as with any other 3D object. The color can be also changed with standard methods in the base class CRenderizable.
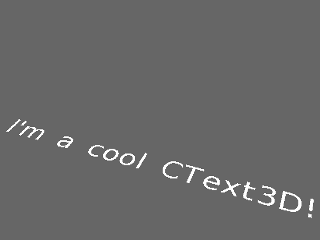
This class is based on code from libcvd (BSD, http://www.edwardrosten.com/cvd/)
See also:
#include <mrpt/opengl/CText3D.h> class CText3D: public mrpt::opengl::CRenderizableShaderText { public: // construction CText3D( const std::string& str = std::string(""), const std::string& fontName = std::string("sans"), const float scale = 1.0, const mrpt::opengl::TOpenGLFontStyle text_style = mrpt::opengl::NICE, const double text_spacing = 1.5, const double text_kerning = 0.1 ); // methods void setString(const std::string& s); const std::string& getString() const; void setFont(const std::string& font); const std::string& getFont() const; void setTextStyle(const mrpt::opengl::TOpenGLFontStyle text_style); mrpt::opengl::TOpenGLFontStyle getTextStyle() const; void setTextSpacing(const double text_spacing); double setTextSpacing() const; void setTextKerning(const double text_kerning); double setTextKerning() const; virtual mrpt::math::TBoundingBox getBoundingBox() const; virtual void toYAMLMap(mrpt::containers::yaml& propertiesMap) const; };
Inherited Members
public: // structs struct RenderContext; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual auto getBoundingBox() const = 0; virtual void freeOpenGLResources() = 0; virtual shader_list_t requiredShaders() const; virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual void onUpdateBuffers_Text() = 0; virtual void freeOpenGLResources();
Methods
void setString(const std::string& s)
Sets the displayed string.
const std::string& getString() const
Returns the currently text associated to this object.
void setFont(const std::string& font)
Changes the font name, among accepted values: “sans”, “mono”, “serif”.
const std::string& getFont() const
Returns the text font.
void setTextStyle(const mrpt::opengl::TOpenGLFontStyle text_style)
Change drawing style: FILL, OUTLINE, NICE.
mrpt::opengl::TOpenGLFontStyle getTextStyle() const
Gets the current drawing style.
virtual mrpt::math::TBoundingBox getBoundingBox() const
Evaluates the bounding box of this object (including possible children) in the coordinate frame of the object parent.
virtual void toYAMLMap(mrpt::containers::yaml& propertiesMap) const
Used from COpenGLScene::asYAML().
(New in MRPT 2.4.2)