class mrpt::opengl::CPointCloudColoured
A cloud of points, each one with an individual colour (R,G,B).
The alpha component is shared by all the points and is stored in the base member m_color_A.
To load from a points-map, CPointCloudColoured::loadFromPointsMap().
This class uses smart optimizations while rendering to efficiently draw clouds of millions of points, using octrees.
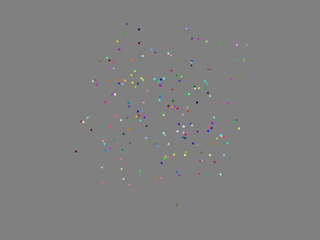
See also:
opengl::COpenGLScene, opengl::CPointCloud
#include <mrpt/opengl/CPointCloudColoured.h> class CPointCloudColoured: public mrpt::opengl::CRenderizableShaderPoints, public mrpt::opengl::COctreePointRenderer, public mrpt::opengl::PLY_Importer, public mrpt::opengl::PLY_Exporter { public: // construction CPointCloudColoured(); // methods void push_back(float x, float y, float z, float R, float G, float B, float A = 1); void insertPoint(const mrpt::math::TPointXYZfRGBAu8& p); void resize(size_t N); void reserve(size_t N); const mrpt::math::TPoint3Df& getPoint3Df(size_t i) const; void setPoint(size_t i, const mrpt::math::TPointXYZfRGBAu8& p); void setPoint_fast(const size_t i, const mrpt::math::TPointXYZfRGBAu8& p); void setPoint_fast(const size_t i, const float x, const float y, const float z); void setPointColor_fast(size_t index, float R, float G, float B, float A = 1); void setPointColor_u8_fast( size_t index, uint8_t r, uint8_t g, uint8_t b, uint8_t a = 0xff ); void getPointColor_fast(size_t index, float& R, float& G, float& B) const; void getPointColor_fast( size_t index, uint8_t& r, uint8_t& g, uint8_t& b ) const; mrpt::img::TColor getPointColor(size_t index) const; size_t size() const; bool empty() const; void clear(); template <class POINTSMAP> void loadFromPointsMap(const POINTSMAP* themap); size_t getActuallyRendered() const; void recolorizeByCoordinate( const float coord_min, const float coord_max, const int coord_index = 2, const mrpt::img::TColormap color_map = mrpt::img::cmJET ); virtual void onUpdateBuffers_Points(); void markAllPointsAsNew(); virtual mrpt::math::TBoundingBox getBoundingBox() const; void render_subset( const bool all, const std::vector<size_t>& idxs, const float render_area_sqpixels ) const; virtual void toYAMLMap(mrpt::containers::yaml& propertiesMap) const; };
Inherited Members
public: // structs struct RenderContext; struct TNode; struct TRenderQueueElement; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual auto getBoundingBox() const = 0; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Points() = 0; size_t octree_get_node_count() const; size_t octree_get_visible_nodes() const; void octree_mark_as_outdated(); void octree_get_graphics_boundingboxes( mrpt::opengl::CSetOfObjects& gl_bb, const float lines_width = 1, const mrpt::img::TColorf& lines_color = mrpt::img::TColorf(1, 1, 1), const bool draw_solid_boxes = false ) const; void octree_debug_dump_tree(std::ostream& o) const;
Methods
void push_back( float x, float y, float z, float R, float G, float B, float A = 1 )
Inserts a new point into the point cloud.
void insertPoint(const mrpt::math::TPointXYZfRGBAu8& p)
inserts a new point
void resize(size_t N)
Set the number of points, with undefined contents.
void reserve(size_t N)
Like STL std::vector’s reserve.
void setPoint(size_t i, const mrpt::math::TPointXYZfRGBAu8& p)
Write an individual point (checks for “i” in the valid range only in Debug).
void setPoint_fast(const size_t i, const mrpt::math::TPointXYZfRGBAu8& p)
Like setPoint() but does not check for index out of bounds.
void setPoint_fast(const size_t i, const float x, const float y, const float z)
Like setPoint() but does not check for index out of bounds.
void setPointColor_fast(size_t index, float R, float G, float B, float A = 1)
Like setPointColor
but without checking for out-of-index erors.
void getPointColor_fast(size_t index, float& R, float& G, float& B) const
Like getPointColor
but without checking for out-of-index erors.
size_t size() const
Return the number of points.
void clear()
Erase all the points.
template <class POINTSMAP> void loadFromPointsMap(const POINTSMAP* themap)
Load the points from any other point map class supported by the adapter mrpt::opengl::PointCloudAdapter.
size_t getActuallyRendered() const
Get the number of elements actually rendered in the last render event.
void recolorizeByCoordinate( const float coord_min, const float coord_max, const int coord_index = 2, const mrpt::img::TColormap color_map = mrpt::img::cmJET )
Regenerates the color of each point according the one coordinate (coord_index:0,1,2 for X,Y,Z) and the given color map.
virtual void onUpdateBuffers_Points()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual mrpt::math::TBoundingBox getBoundingBox() const
Evaluates the bounding box of this object (including possible children) in the coordinate frame of the object parent.
void render_subset( const bool all, const std::vector<size_t>& idxs, const float render_area_sqpixels ) const
Render a subset of points (required by octree renderer)
virtual void toYAMLMap(mrpt::containers::yaml& propertiesMap) const
Used from COpenGLScene::asYAML().
(New in MRPT 2.4.2)