class mrpt::opengl::CGridPlaneXZ
A grid of lines over the XZ plane.
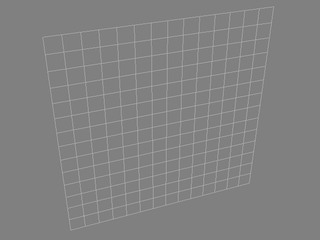
See also:
#include <mrpt/opengl/CGridPlaneXZ.h> class CGridPlaneXZ: public mrpt::opengl::CRenderizableShaderWireFrame { public: // construction CGridPlaneXZ( float xMin = -10, float xMax = 10, float zMin = -10, float zMax = 10, float y = 0, float frequency = 1, float lineWidth = 1.3f, bool antiAliasing = true ); // methods void setPlaneLimits( float xmin, float xmax, float zmin, float zmax ); void getPlaneLimits( float& xmin, float& xmax, float& zmin, float& zmax ) const; void setPlaneYcoord(float y); float getPlaneYcoord() const; void setGridFrequency(float freq); float getGridFrequency() const; virtual void onUpdateBuffers_Wireframe(); virtual mrpt::math::TBoundingBox getBoundingBox() const; };
Inherited Members
public: // structs struct RenderContext; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual auto getBoundingBox() const = 0; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Wireframe() = 0;
Construction
CGridPlaneXZ( float xMin = -10, float xMax = 10, float zMin = -10, float zMax = 10, float y = 0, float frequency = 1, float lineWidth = 1.3f, bool antiAliasing = true )
Constructor.
Methods
virtual void onUpdateBuffers_Wireframe()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual mrpt::math::TBoundingBox getBoundingBox() const
Evaluates the bounding box of this object (including possible children) in the coordinate frame of the object parent.