class mrpt::poses::CPoint3D
A class used to store a 3D point.
For a complete description of Points/Poses, see mrpt::poses::CPoseOrPoint, or refer to the 2D/3D Geometry tutorial in the wiki.
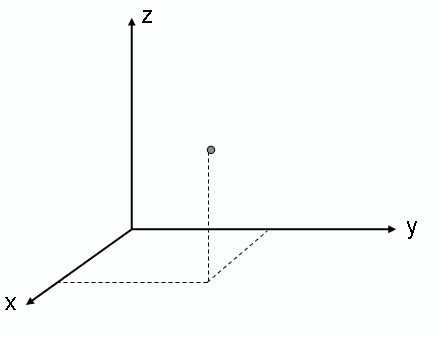
See also:
#include <mrpt/poses/CPoint3D.h> class CPoint3D: public mrpt::poses::CPoint, public mrpt::serialization::CSerializable { public: // typedefs typedef double value_type; typedef double& reference; typedef double const_reference; typedef std::size_t size_type; typedef std::ptrdiff_t difference_type; // enums enum { is_3D_val = 1, }; enum { is_PDF_val = 0, }; enum { static_size = 3, }; // fields mrpt::math::CVectorFixedDouble<3> m_coords; // construction CPoint3D(const double x = 0, const double y = 0, const double z = 0); CPoint3D(const mrpt::math::CVectorFixedDouble<3>& xyz); CPoint3D(const CPoint2D& p); CPoint3D(const CPose3D& p); CPoint3D(const CPose2D& p); CPoint3D(const mrpt::math::TPoint3D& p); // methods static constexpr size_type size(); static constexpr bool empty(); static constexpr size_type max_size(); static void resize(const size_t n); double operator [] (unsigned int i) const; double& operator [] (unsigned int i); mrpt::math::TPoint3D asTPoint() const; CPoint3D operator - (const CPose3D& b) const; CPoint3D operator - (const CPoint3D& b) const; CPoint3D operator + (const CPoint3D& b) const; CPose3D operator + (const CPose3D& b) const; void asVector(vector_t& v) const; virtual void setToNaN(); static constexpr bool is_3D(); static constexpr bool is_PDF(); };
Inherited Members
public: // typedefs typedef mrpt::math::CVectorFixedDouble<DIM> vector_t; // methods double& x(); double& y(); void x(const double v); void y(const double v); void x_incr(const double v); void y_incr(const double v); const DERIVEDCLASS& derived() const; DERIVEDCLASS& derived(); template <class OTHERCLASS> void AddComponents(const OTHERCLASS& b); void operator *= (const double s); template <class MATRIX44> void getHomogeneousMatrix(MATRIX44& out_HM) const; virtual std::string asString() const; void fromString(const std::string& s); double x() const; double y() const; template <class OTHERCLASS, std::size_t DIM2> double sqrDistanceTo(const CPoseOrPoint<OTHERCLASS, DIM2>& b) const; template <class OTHERCLASS, std::size_t DIM2> double distanceTo(const CPoseOrPoint<OTHERCLASS, DIM2>& b) const; double distanceTo(const mrpt::math::TPoint3D& b) const; double distance2DToSquare(double ax, double ay) const; double distance3DToSquare(double ax, double ay, double az) const; double distance2DTo(double ax, double ay) const; double distance3DTo(double ax, double ay, double az) const; double norm() const; vector_t asVectorVal() const; template <class MATRIX44> MATRIX44 getHomogeneousMatrixVal() const; template <class MATRIX44> void getInverseHomogeneousMatrix(MATRIX44& out_HM) const; template <class MATRIX44> MATRIX44 getInverseHomogeneousMatrixVal() const; virtual void setToNaN() = 0; static bool is3DPoseOrPoint();
Typedefs
typedef double value_type
The type of the elements.
Fields
mrpt::math::CVectorFixedDouble<3> m_coords
[x,y,z]
Construction
CPoint3D(const double x = 0, const double y = 0, const double z = 0)
Constructor for initializing point coordinates.
CPoint3D(const mrpt::math::CVectorFixedDouble<3>& xyz)
Constructor from a XYZ 3-vector.
CPoint3D(const CPoint2D& p)
Constructor from an CPoint2D object.
CPoint3D(const CPose3D& p)
Constructor from an CPose3D object.
CPoint3D(const CPose2D& p)
Constructor from an CPose2D object.
CPoint3D(const mrpt::math::TPoint3D& p)
Constructor from lightweight object.
Methods
CPoint3D operator - (const CPose3D& b) const
Returns this point as seen from “b”, i.e.
result = this - b
CPoint3D operator - (const CPoint3D& b) const
Returns this point minus point “b”, i.e.
result = this - b
CPoint3D operator + (const CPoint3D& b) const
Returns this point plus point “b”, i.e.
result = this + b
CPose3D operator + (const CPose3D& b) const
Returns this point plus pose “b”, i.e.
result = this + b
void asVector(vector_t& v) const
Return the pose or point as a 3x1 vector [x y z]’.
virtual void setToNaN()
Set all data fields to quiet NaN.