class mrpt::hwdrivers::CNTRIPEmitter
This “virtual driver” encapsulates a NTRIP client (see CNTRIPClient) but adds the functionality of dumping the received datastream to a given serial port.
Used within rawlog-grabber, along CGPSInterface, this class allows to build a powerful & simple RTK-capable GPS receiver system.
Therefore, this sensor will never “collect” any observation via the CGenericSensor interface.
See also the example configuration file for rawlog-grabber in “share/mrpt/config_files/rawlog-grabber”.
PARAMETERS IN THE ".INI"-LIKE CONFIGURATION STRINGS: ------------------------------------------------------- [supplied_section_name] COM_port_WIN = COM1 // Serial port where the NTRIP stream will be dumped to. COM_port_LIN = ttyUSB0 baudRate = 38400 #transmit_to_server = true // (Default:true) Whether to send back to the TCP/IP caster all data received from the output serial port #raw_output_file_prefix = raw_ntrip_data // If provided, save raw data from the NTRIP to a file, useful for post-processing. In this case, not having a serial port configured (commented out) is a valid configuration. server = 143.123.9.129 // NTRIP caster IP port = 2101 mountpoint = MYPOINT23 //user = pepe // User & password optional. //password = loco
The next picture summarizes existing MRPT classes related to GPS / GNSS devices (CGPSInterface, CNTRIPEmitter, CGPS_NTRIP):
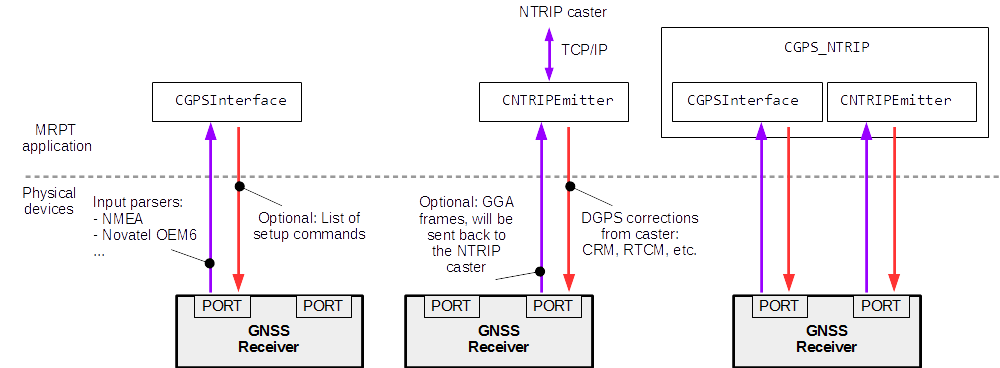
See also:
CGPSInterface, CGPS_NTRIP, CNTRIPClient
#include <mrpt/hwdrivers/CNTRIPEmitter.h> class CNTRIPEmitter: public mrpt::hwdrivers::CGenericSensor { public: // construction CNTRIPEmitter(); // methods void setOutputSerialPort(const std::string& port); std::string getOutputSerialPort() const; void setRawOutputFilePrefix(const std::string& outfile); std::string getRawOutputFilePrefix() const; virtual void initialize(); virtual void doProcess(); CNTRIPClient& getNTRIPClient(); const CNTRIPClient& getNTRIPClient() const; };
Inherited Members
public: // methods CGenericSensor& operator = (const CGenericSensor&); virtual void doProcess() = 0;
Construction
CNTRIPEmitter()
Constructor.
Methods
void setOutputSerialPort(const std::string& port)
Changes the serial port to connect to (call prior to ‘doProcess’), for example “COM1” or “ttyS0”.
This is not needed if the configuration is loaded with “loadConfig”.
virtual void initialize()
Set up the NTRIP communications, raising an exception on fatal errors.
Called automatically by rawlog-grabber. If used manually, call after “loadConfig” and before “doProcess”.
virtual void doProcess()
The main loop, which must be called in a timely fashion in order to process the incomming NTRIP data stream and dump it to the serial port.
This method is called automatically when used within rawlog-grabber.
CNTRIPClient& getNTRIPClient()
Exposes the NTRIP client object.
const CNTRIPClient& getNTRIPClient() const
Exposes the NTRIP client object.