class mrpt::gui::CDisplayWindowGUI
A window with powerful GUI capabilities, via the nanogui library.
You can add a background mrpt::opengl::COpenGLScene object rendered on the background of the entire window by setting an object in field background_scene
, locking its mutex background_scene_mtx
.
Refer to nanogui API docs or MRPT examples for further usage examples. A typical lifecycle of a GUI app with this class might look like:
nanogui::init(); { mrpt::gui::CDisplayWindowGUI win; // Populate win adding UI controls, etc. // ... win.performLayout(); win.drawAll(); win.setVisible(true); nanogui::mainloop(); } nanogui::shutdown();
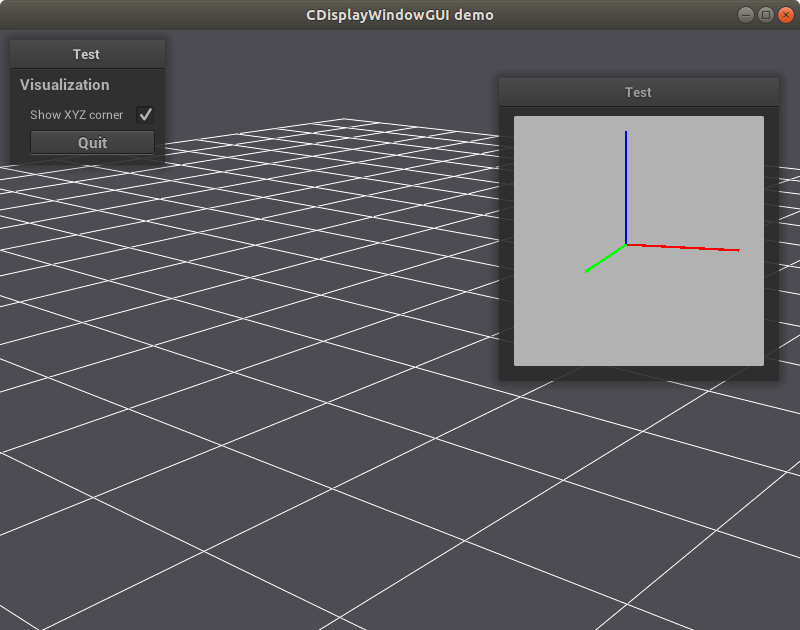
Create managed subwindows with createManagedSubWindow(), with built-in support for minimize and restore. See demo video in: https://www.youtube.com/watch?v=QKMzdlZRW50
#include <mrpt/gui/CDisplayWindowGUI.h> class CDisplayWindowGUI: public Screen { public: // typedefs typedef std::function<void(void)> loop_callback_t; typedef std::function<bool(const std::vector<std::string>&)> drop_files_callback_t; typedef std::function<bool(int, int, int, int)> keyboard_callback_t; typedef std::shared_ptr<CDisplayWindowGUI> Ptr; typedef std::shared_ptr<const CDisplayWindowGUI> ConstPtr; // structs struct SubWindows; // classes class SubWindow; // fields mrpt::opengl::COpenGLScene::Ptr background_scene; std::mutex background_scene_mtx; // construction CDisplayWindowGUI( const std::string& caption = std::string(), unsigned int width = 400, unsigned int height = 300, const CDisplayWindowGUI_Params& p = CDisplayWindowGUI_Params() ); // methods void resize(unsigned int width, unsigned int height); void setPos(int x, int y); void setWindowTitle(const std::string& str); void setIcon(const mrpt::img::CImage& img); void setIconFromData( const char* imgData, unsigned int width, unsigned int height, const uint8_t transparent ); void addLoopCallback(const loop_callback_t& callback); const auto& loopCallbacks() const; void addDropFilesCallback(const drop_files_callback_t& callback); const auto& dropFilesCallbacks() const; void addKeyboardCallback(const keyboard_callback_t& callback); const auto& keyboardCallbacks() const; void setLoopCallback(const loop_callback_t& callback); loop_callback_t loopCallback() const; void setDropFilesCallback(const drop_files_callback_t& callback); drop_files_callback_t dropFilesCallback() const; void setKeyboardCallback(const keyboard_callback_t& callback); keyboard_callback_t keyboardCallback() const; template <typename... Args> static Ptr Create(Args&&... args); nanogui::Window* createManagedSubWindow(const std::string& title); nanogui::Window* getSubWindowsUI(); const nanogui::Window* getSubWindowsUI() const; const nanogui::Window* getSubwindow(size_t index) const; size_t getSubwindowCount() const; void subwindowMinimize(size_t index); void subwindowRestore(size_t index); void subwindowSetFocused(size_t index); CGlCanvasBase& camera(); const CGlCanvasBase& camera() const; nanogui::Screen* nanogui_screen(); virtual void drawContents(); virtual void onIdleLoopTasks(); };
Construction
CDisplayWindowGUI( const std::string& caption = std::string(), unsigned int width = 400, unsigned int height = 300, const CDisplayWindowGUI_Params& p = CDisplayWindowGUI_Params() )
Regular ctor from caption and size.
Methods
void resize(unsigned int width, unsigned int height)
Resizes the window.
void setPos(int x, int y)
Changes the position of the window on the screen.
void setWindowTitle(const std::string& str)
Changes the window title.
void setIcon(const mrpt::img::CImage& img)
Sets the window icon, which must must either RGB or (preferred) RGBA channels.
You can read it from a .png or .ico file using mrpt::img::CImage::loadFromFile().
The image will be resized as needed. Good sizes include 16x16, 32x32 and 48x48.
(New in MRPT 2.4.2)
void addLoopCallback(const loop_callback_t& callback)
Every time the window is about to be repainted, an optional callback can be called, if provided via this method.
This method can be safely called multiple times to register multiple callbacks.
(New in MRPT 2.3.2)
void addDropFilesCallback(const drop_files_callback_t& callback)
Handles drag-and drop events of files into the window.
This method can be safely called multiple times to register multiple callbacks.
(New in MRPT 2.3.2)
void addKeyboardCallback(const keyboard_callback_t& callback)
Handles keyboard events.
This method can be safely called multiple times to register multiple callbacks.
(New in MRPT 2.3.2)
void setLoopCallback(const loop_callback_t& callback)
This call replaces all existing loop callbacks. See addLoopCallback().
Deprecated In MRPT 2.3.2, replaced by addLoopCallback()
loop_callback_t loopCallback() const
Deprecated In MRPT 2.3.2, replaced by loopCallbacks()
void setDropFilesCallback(const drop_files_callback_t& callback)
This call replaces all existing drop file callbacks. See addDropFilesCallback().
Deprecated In MRPT 2.3.2, replaced by addDropFilesCallback()
drop_files_callback_t dropFilesCallback() const
Deprecated In MRPT 2.3.2, replaced by dropFilesCallbacks()
void setKeyboardCallback(const keyboard_callback_t& callback)
This call replaces all existing keyboard callbacks. See addKeyboardCallback().
Deprecated In MRPT 2.3.2, replaced by addKeyboardCallback()
keyboard_callback_t keyboardCallback() const
Deprecated In MRPT 2.3.2, replaced by keyboardCallbacks()
template <typename... Args> static Ptr Create(Args&&... args)
Class factory returning a smart pointer, equivalent to std::make_shared<>(...)
nanogui::Window* createManagedSubWindow(const std::string& title)
Creates and return a nanogui::Window, adds to it basic minimize/restore tool buttons and add it to the list of handled subwindows so it gets listed in the subwindows control UI.
User should set a layout manager, width, height, etc. in the returned window as desired.
The returned object is owned by the nanogui system, you should NOT delete it.
The first time this is called, an additional subWindow will be created (default position:bottom left corner) to hold minimized windows. You can access and modify this windows via getSubWindowsUI().
[New in MRPT 2.1.1]
const nanogui::Window* getSubwindow(size_t index) const
Direct (read-only) access to managed subwindows by 0-based index (creation order).
[New in MRPT 2.3.1]
See also:
size_t getSubwindowCount() const
Get the number of managed subwindows.
[New in MRPT 2.3.1]
See also:
void subwindowMinimize(size_t index)
Minimize a subwindow.
[New in MRPT 2.3.1]
void subwindowRestore(size_t index)
Restore a minimized subwindow.
[New in MRPT 2.3.1]
void subwindowSetFocused(size_t index)
Forces focus on a subwindow.
[New in MRPT 2.3.1]