class mrpt::opengl::CSphere
A solid or wire-frame sphere.
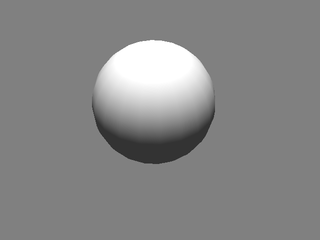
See also:
#include <mrpt/opengl/CSphere.h> class CSphere: public mrpt::opengl::CGeneralizedEllipsoidTemplate { public: // typedefs typedef mrpt::math::CMatrixFixed<float, DIM, 1> array_parameter_t; typedef mrpt::math::CMatrixFixed<float, DIM, 1> array_point_t; // construction CSphere(float radius = 1.0f, int nDivsLongitude = 20, int nDivsLatitude = 20); // methods virtual void renderUpdateBuffers() const; void setRadius(float r); float getRadius() const; void setNumberDivsLongitude(int N); void setNumberDivsLatitude(int N); virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const; virtual mrpt::math::TBoundingBox getBoundingBox() const; uint32_t getNumberOfSegments(); bool isAntiAliasingEnabled() const; };
Inherited Members
public: // structs struct RenderContext; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual auto getBoundingBox() const = 0; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Triangles() = 0; virtual void onUpdateBuffers_Wireframe() = 0;
Construction
CSphere(float radius = 1.0f, int nDivsLongitude = 20, int nDivsLatitude = 20)
Constructor.
Methods
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const
Simulation of ray-trace, given a pose.
Returns true if the ray effectively collisions with the object (returning the distance to the origin of the ray in “dist”), or false in other case. “dist” variable yields undefined behaviour when false is returned
virtual mrpt::math::TBoundingBox getBoundingBox() const
Evaluates the bounding box of this object (including possible children) in the coordinate frame of the object parent.