class mrpt::gui::CDisplayWindow
This class creates a window as a graphical user interface (GUI) for displaying images to the user.
For a list of supported events with the observer/observable pattern, see the discussion in mrpt::gui::CBaseGUIWindow.
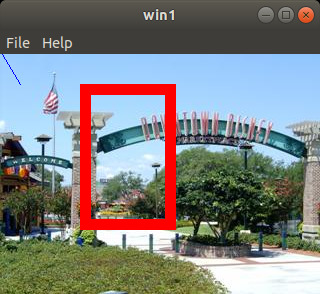
#include <mrpt/gui/CDisplayWindow.h> class CDisplayWindow: public mrpt::gui::CBaseGUIWindow { public: // typedefs typedef std::shared_ptr<CDisplayWindow> Ptr; typedef std::shared_ptr<const CDisplayWindow> ConstPtr; // construction CDisplayWindow( const std::string& windowCaption = std::string(), unsigned int initWidth = 400, unsigned int initHeight = 400 ); // methods virtual bool getLastMousePosition(int& x, int& y) const; virtual void setCursorCross(bool cursorIsCross); void showImageAndPoints( const mrpt::img::CImage& img, const mrpt::math::CVectorFloat& x, const mrpt::math::CVectorFloat& y, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showNumbers = false ); void showImageAndPoints( const mrpt::img::CImage& img, const std::vector<float>& x, const std::vector<float>& y, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showNumbers = false ); template <class FEATURELIST> void showImageAndPoints( const mrpt::img::CImage& img, const FEATURELIST& list, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showIDs = false ); template <class FEATURELIST> void showTiledImageAndPoints( const mrpt::img::CImage& img, const FEATURELIST& list, const mrpt::img::TColor& color = mrpt::img::TColor::red() ); template <class MATCHEDLIST> void showImagesAndMatchedPoints( const mrpt::img::CImage& img1, const mrpt::img::CImage& img2, const MATCHEDLIST& mList, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showNumbers = false ); template <class FEATURELIST> void showImagesAndMatchedPoints( const mrpt::img::CImage& img1, const mrpt::img::CImage& img2, const FEATURELIST& leftList, const FEATURELIST& rightList, const mrpt::img::TColor& color = mrpt::img::TColor::red() ); void showImage(const mrpt::img::CImage& img); void plot(const mrpt::math::CVectorFloat& x, const mrpt::math::CVectorFloat& y); void plot(const mrpt::math::CVectorFloat& y); virtual void resize(unsigned int width, unsigned int height); virtual void setPos(int x, int y); void enableCursorCoordinatesVisualization(bool enable); virtual void setWindowTitle(const std::string& str); static CDisplayWindow::Ptr Create( const std::string& windowCaption, unsigned int initWidth = 400, unsigned int initHeight = 400 ); };
Inherited Members
public: // methods void* getWxObject(); void notifyChildWindowDestruction(); void notifySemThreadReady(); bool isOpen(); virtual void resize(unsigned int width, unsigned int height) = 0; virtual void setPos(int x, int y) = 0; virtual void setWindowTitle(const std::string& str) = 0; virtual bool getLastMousePosition(int& x, int& y) const = 0; virtual void setCursorCross(bool cursorIsCross) = 0; int waitForKey(bool ignoreControlKeys = true, mrptKeyModifier* out_pushModifier = nullptr); bool keyHit() const; void clearKeyHitFlag(); int getPushedKey(mrptKeyModifier* out_pushModifier = nullptr);
Construction
CDisplayWindow( const std::string& windowCaption = std::string(), unsigned int initWidth = 400, unsigned int initHeight = 400 )
Constructor.
Methods
virtual bool getLastMousePosition(int& x, int& y) const
Gets the last x,y pixel coordinates of the mouse.
Returns:
False if the window is closed.
virtual void setCursorCross(bool cursorIsCross)
Set cursor style to default (cursorIsCross=false) or to a cross (cursorIsCross=true)
void showImageAndPoints( const mrpt::img::CImage& img, const mrpt::math::CVectorFloat& x, const mrpt::math::CVectorFloat& y, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showNumbers = false )
Show a given color or grayscale image on the window and print a set of points on it.
It adapts the size of the window to that of the image.
void showImageAndPoints( const mrpt::img::CImage& img, const std::vector<float>& x, const std::vector<float>& y, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showNumbers = false )
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template <class FEATURELIST> void showImageAndPoints( const mrpt::img::CImage& img, const FEATURELIST& list, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showIDs = false )
Show a given color or grayscale image on the window and print a set of points on it.
It adapts the size of the window to that of the image. The class of FEATURELIST can be: mrpt::vision::CFeatureList or any STL container of entities having “x”,”y” and “ID” fields.
template <class FEATURELIST> void showTiledImageAndPoints( const mrpt::img::CImage& img, const FEATURELIST& list, const mrpt::img::TColor& color = mrpt::img::TColor::red() )
Show a given color or grayscale image on the window and print a set of points on it and a set of lines splitting the image in tiles.
It adapts the size of the window to that of the image. The class of FEATURELIST can be: mrpt::vision::CFeatureList
template <class MATCHEDLIST> void showImagesAndMatchedPoints( const mrpt::img::CImage& img1, const mrpt::img::CImage& img2, const MATCHEDLIST& mList, const mrpt::img::TColor& color = mrpt::img::TColor::red(), bool showNumbers = false )
Show a pair of given color or grayscale images (put together) on the window and print a set of matches on them.
It adapts the size of the window to that of the image. MATCHEDLIST can be of the class: mrpt::vision::CMatchedFeatureList, or any STL container of pairs of anything having “.x” and “.y” (e.g. mrpt::math::TPoint2D)
template <class FEATURELIST> void showImagesAndMatchedPoints( const mrpt::img::CImage& img1, const mrpt::img::CImage& img2, const FEATURELIST& leftList, const FEATURELIST& rightList, const mrpt::img::TColor& color = mrpt::img::TColor::red() )
Show a pair of given color or grayscale images (put together) on the window and print a set of matches on them.
It adapts the size of the window to that of the image. FEATURELIST can be of the class: mrpt::vision::CFeatureList
void showImage(const mrpt::img::CImage& img)
Show a given color or grayscale image on the window.
It adapts the size of the window to that of the image.
void plot(const mrpt::math::CVectorFloat& x, const mrpt::math::CVectorFloat& y)
Plots a graph in MATLAB-like style.
void plot(const mrpt::math::CVectorFloat& y)
Plots a graph in MATLAB-like style.
virtual void resize(unsigned int width, unsigned int height)
Resizes the window, stretching the image to fit into the display area.
virtual void setPos(int x, int y)
Changes the position of the window on the screen.
void enableCursorCoordinatesVisualization(bool enable)
Enables or disables the visualization of cursor coordinates on the window caption (default = enabled).
virtual void setWindowTitle(const std::string& str)
Changes the window title text.
static CDisplayWindow::Ptr Create( const std::string& windowCaption, unsigned int initWidth = 400, unsigned int initHeight = 400 )
Class factory returning a smart pointer, equivalent to std::make_shared<>(...)