class mrpt::poses::CPoint2D¶
A class used to store a 2D point.
For a complete description of Points/Poses, see mrpt::poses::CPoseOrPoint, or refer to the 2D/3D Geometry tutorial in the wiki.
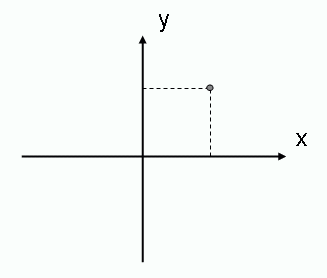
See also:
#include <mrpt/poses/CPoint2D.h> class CPoint2D: public mrpt::poses::CPoint, public mrpt::serialization::CSerializable { public: // typedefs typedef double value_type; typedef double& reference; typedef double const_reference; typedef std::size_t size_type; typedef std::ptrdiff_t difference_type; // enums enum { is_3D_val = 0, }; enum { is_PDF_val = 0, }; enum { static_size = 2, }; // fields mrpt::math::CVectorFixedDouble<2> m_coords; // construction CPoint2D(double x = 0, double y = 0); template <class OTHERCLASS, std::size_t DIM2> CPoint2D(const CPoseOrPoint<OTHERCLASS, DIM2>& b); CPoint2D(const mrpt::math::TPoint2D& o); CPoint2D(const mrpt::math::TPoint3D& o); // methods static constexpr size_type size(); static constexpr bool empty(); static constexpr size_type max_size(); static void resize(const size_t n); mrpt::math::TPoint2D asTPoint() const; void asVector(vector_t& v) const; CPoint2D operator - (const CPose2D& b) const; virtual void setToNaN(); static constexpr bool is_3D(); static constexpr bool is_PDF(); };
Inherited Members¶
public: // typedefs typedef mrpt::math::CVectorFixedDouble<DIM> vector_t; // methods double& x(); double& y(); void x(const double v); void y(const double v); void x_incr(const double v); void y_incr(const double v); const DERIVEDCLASS& derived() const; DERIVEDCLASS& derived(); template <class OTHERCLASS> void AddComponents(const OTHERCLASS& b); void operator *= (const double s); template <class MATRIX44> void getHomogeneousMatrix(MATRIX44& out_HM) const; void asString(std::string& s) const; void fromString(const std::string& s); double x() const; double y() const; template <class OTHERCLASS, std::size_t DIM2> double sqrDistanceTo(const CPoseOrPoint<OTHERCLASS, DIM2>& b) const; template <class OTHERCLASS, std::size_t DIM2> double distanceTo(const CPoseOrPoint<OTHERCLASS, DIM2>& b) const; double distanceTo(const mrpt::math::TPoint3D& b) const; double distance2DToSquare(double ax, double ay) const; double distance3DToSquare(double ax, double ay, double az) const; double distance2DTo(double ax, double ay) const; double distance3DTo(double ax, double ay, double az) const; double norm() const; vector_t asVectorVal() const; template <class MATRIX44> MATRIX44 getHomogeneousMatrixVal() const; template <class MATRIX44> void getInverseHomogeneousMatrix(MATRIX44& out_HM) const; template <class MATRIX44> MATRIX44 getInverseHomogeneousMatrixVal() const; virtual void setToNaN() = 0; static bool is3DPoseOrPoint();
Construction¶
CPoint2D(double x = 0, double y = 0)
Constructor for initializing point coordinates.
template <class OTHERCLASS, std::size_t DIM2> CPoint2D(const CPoseOrPoint<OTHERCLASS, DIM2>& b)
Constructor from x/y coordinates given from other pose.
CPoint2D(const mrpt::math::TPoint2D& o)
Implicit constructor from lightweight type.
CPoint2D(const mrpt::math::TPoint3D& o)
Explicit constructor from lightweight type (loses the z coord).
Methods¶
void asVector(vector_t& v) const
Return the pose or point as a 2x1 vector [x, y]’.
CPoint2D operator - (const CPose2D& b) const
The operator D=”this”-b is the pose inverse compounding operator, the resulting points “D” fulfils: “this” = b + D, so that: b == a + (b-a)
virtual void setToNaN()
Set all data fields to quiet NaN.